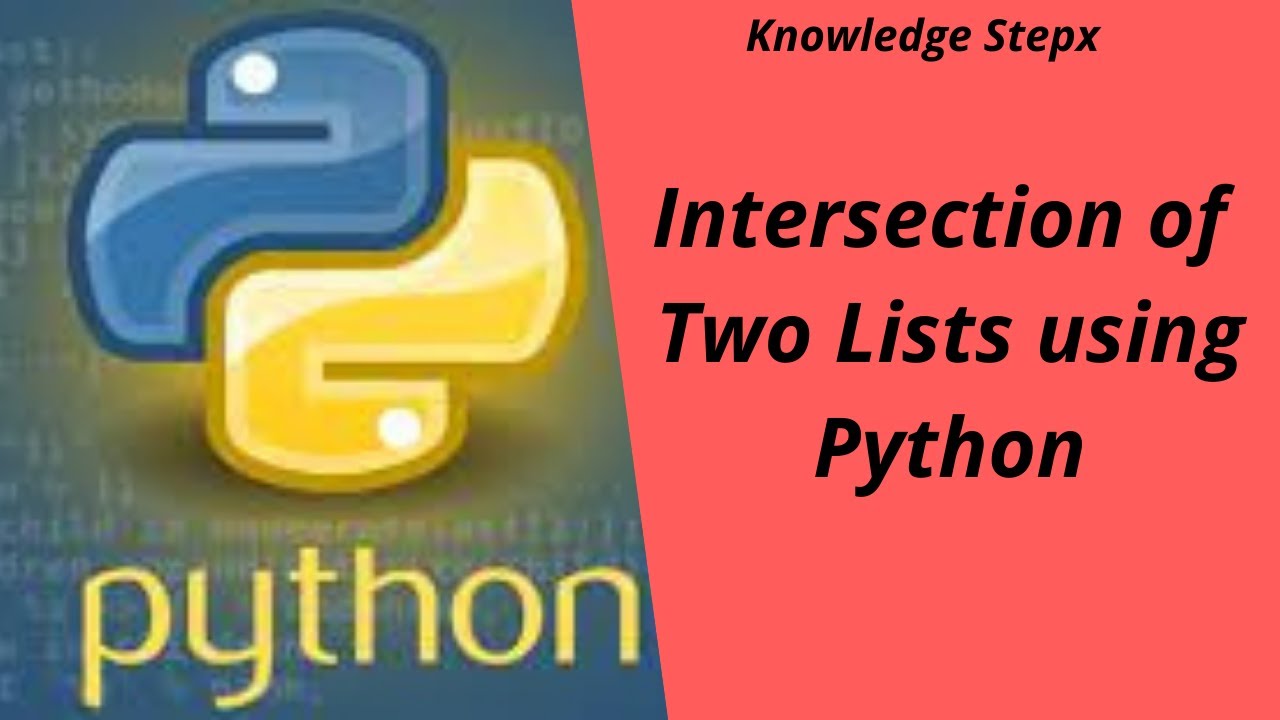
Intersection of two lists using Python YouTube
Python list doesn't have any inbuilt method to do the intersection, so we are converting the list to a set. After the list is converted to a set, we can easily calculate the intersection by using the & operator. Then, we can convert the final set to a list by using list () method. Python program :
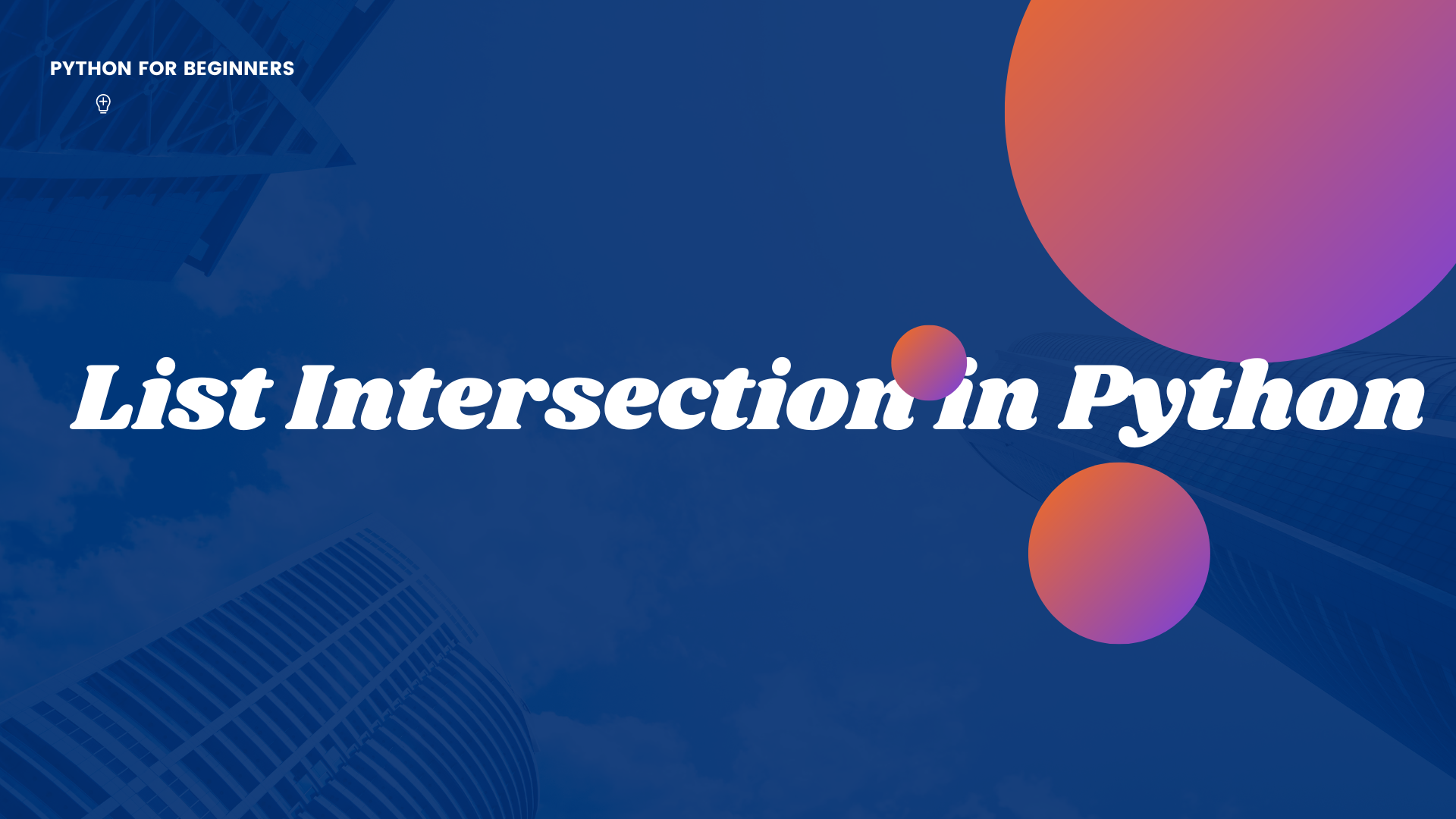
Intersection of Lists in Python
Intersection of two list means we need to take all those elements which are common to both of the initial lists and store them into another list. Now there are various ways in Python, through which we can perform the Intersection of the lists. Examples:
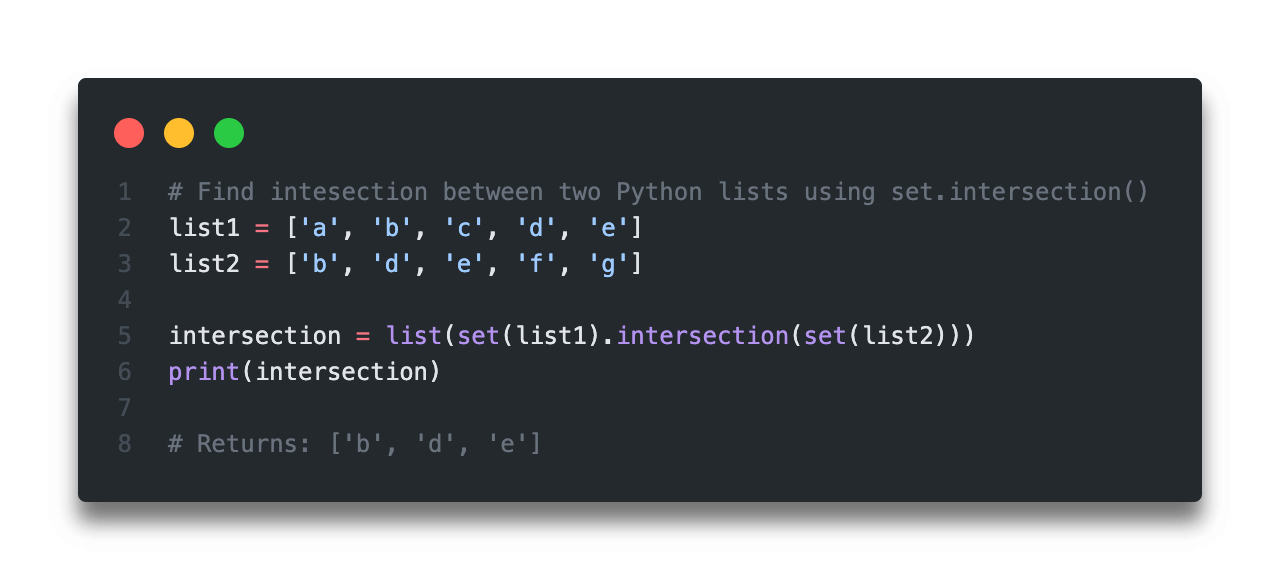
Python Intersection Between Two Lists โข datagy
The intersection of two lists implies identifying the elements that are shared by both lists. Python provides numerous techniques to find this intersection. Utilizing the built-in intersection () function from the collections module is the simplest way to accomplish this.
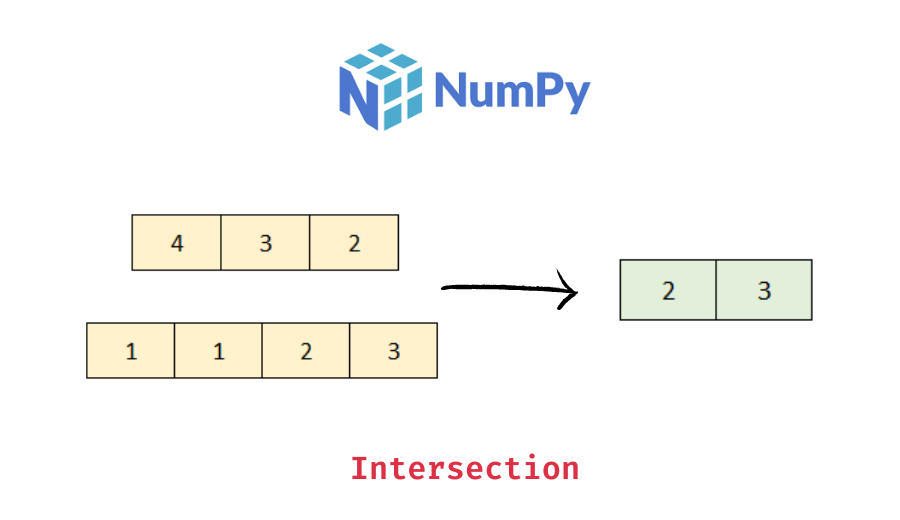
Python Get Intersection of Two Numpy Arrays Data Science Parichay
To perform the intersection of two lists in Python, we just have to create an output list that should contain elements that are present in both the input lists. For instance, if we have list1= [1,2,3,4,5,6] and list2= [2,4,6,8,10,12], the intersection of list1 and list2 will be [2,4,6].
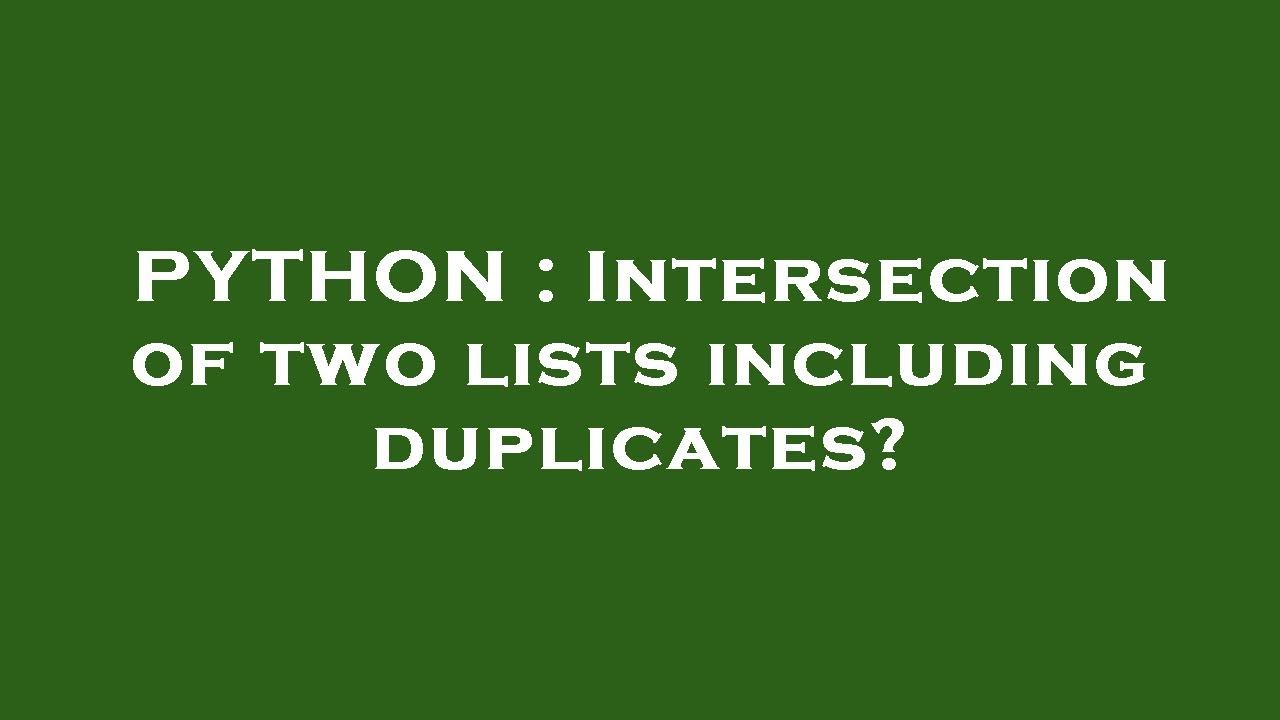
PYTHON Intersection of two lists including duplicates? YouTube
How to find list intersection? [duplicate] Ask Question Asked 13 years, 4 months ago Modified 9 months ago Viewed 525k times 440 This question already has answers here : Common elements comparison between 2 lists (14 answers) Closed 10 months ago. a = [1,2,3,4,5] b = [1,3,5,6] c = a and b print c actual output: [1,3,5,6] expected output: [1,3,5]
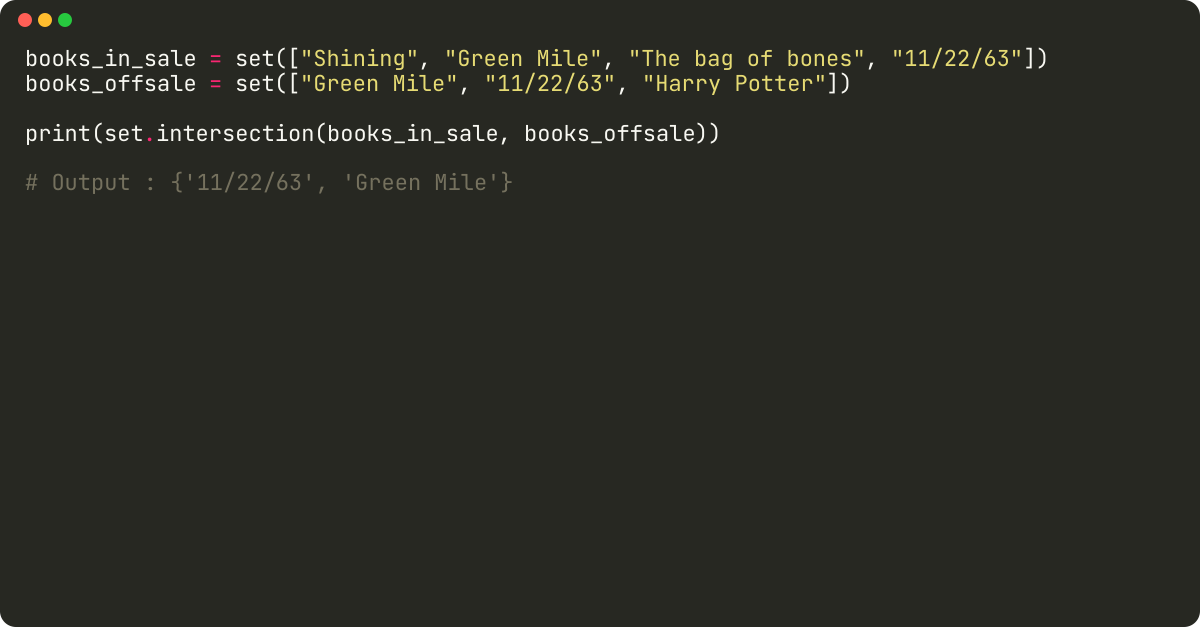
How to get intersection of two lists in python?
Intersection means finding the common elements between two lists. Typically, intersection is set-based, meaning that the values are unduplicated. You will learn some naive methods to find intersection between two Python lists, including for loops and list comprehensions, using the set .intersection () method and using numpy.
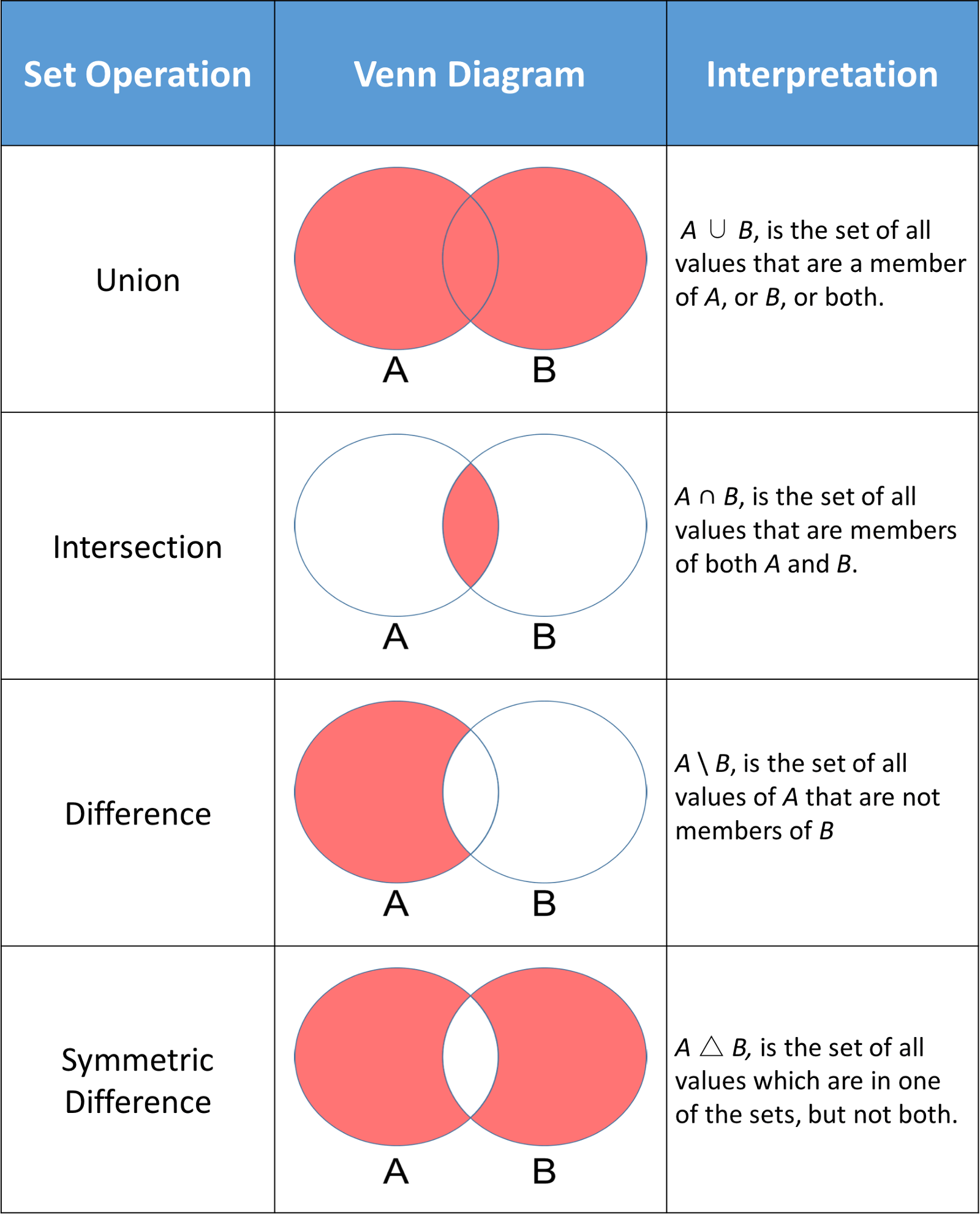
Tutorial) Python Sets vs Lists, and Set Theory DataCamp
Method 4: Using filter () and lambda function. Convert both nested lists into sets using map () and set (). Create a lambda function that takes a nested list and returns True if it's a subset of the second set. Use filter () with the lambda function to get a filter object of the subsets.
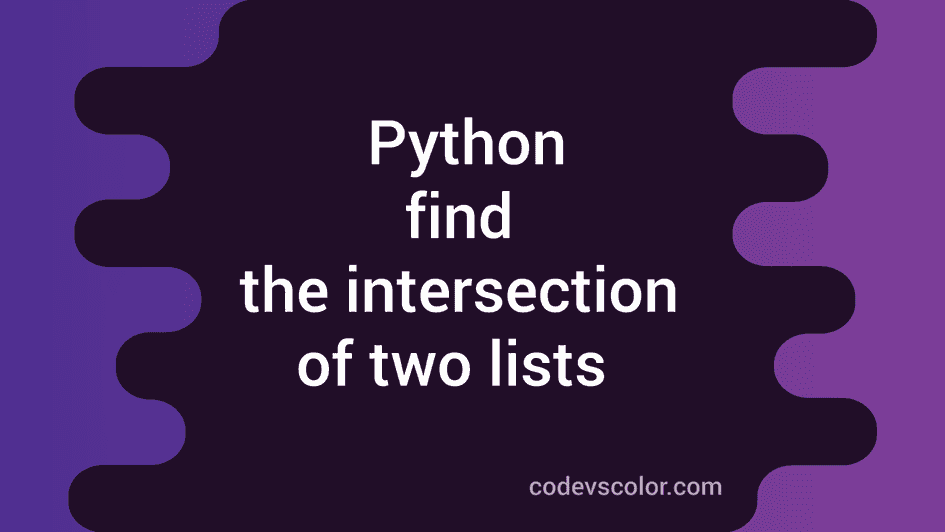
How to find the intersection of two list elements in python CodeVsColor
There are three main methods to find the intersection of two lists in Python: Using sets; Using list comprehension; Using the built-in filter() function; This post explains the code for each method and discusses various factors to consider when deciding which method to use.

Python List Interpolation Intersection of Two Lists YouTube
Basic Equality Check. The most straightforward way to compare two lists is to check for equality. This means ensuring that both lists contain the same elements in the same order. Here's how you can perform a basic equality check: seq1 = [11, 21, 31, 41, 51] seq2 = [11, 21, 31, 41, 51] if seq1 == seq2: print ("The lists are equal.") else.
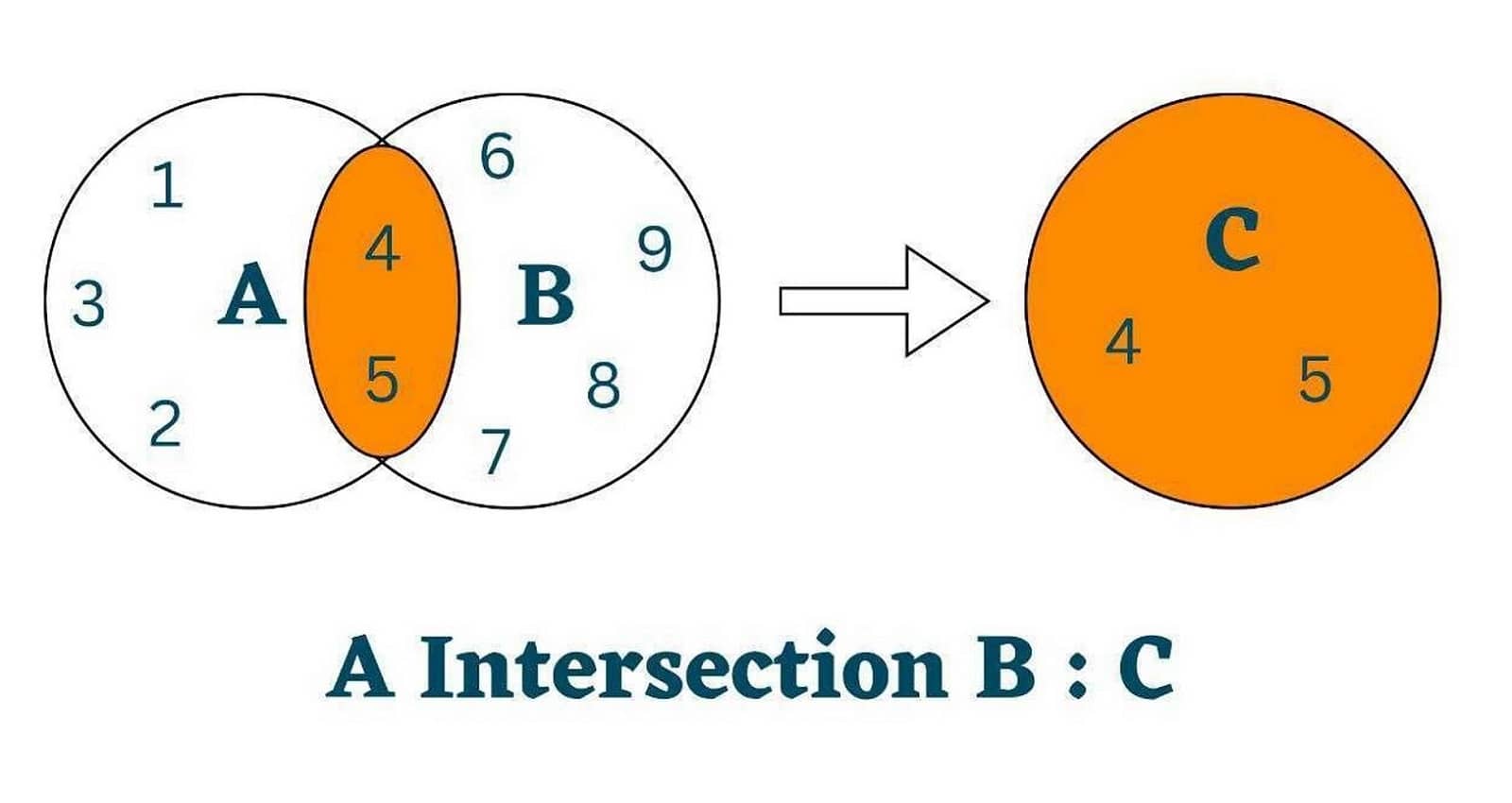
How to Find Intersection of Two Lists in Python? (with code)
Our goal is to develop an algorithm that can efficiently identify the node at which the two lists intersect. Let's see an example. Input: List 1 = [1,3,1,2,4], List 2 = [1,3,2,4] Output: 2. As we can see both the linked lists intersect at node 2. In the simple brute-force approach, we will iterate through one of the lists and check if each.

Python Program to Find the Intersection of Two Lists YouTube
In Python, the easiest way to get the intersection of two lists is by using list comprehension to identify the values which are in both lists. list_1 = [5,3,8,2,1] list_2 = [9,3,4,2,1] intersection_of_lists = [x for x in list_1 if x in list_2] print (intersection_of_lists) #Output: [3, 2, 1]
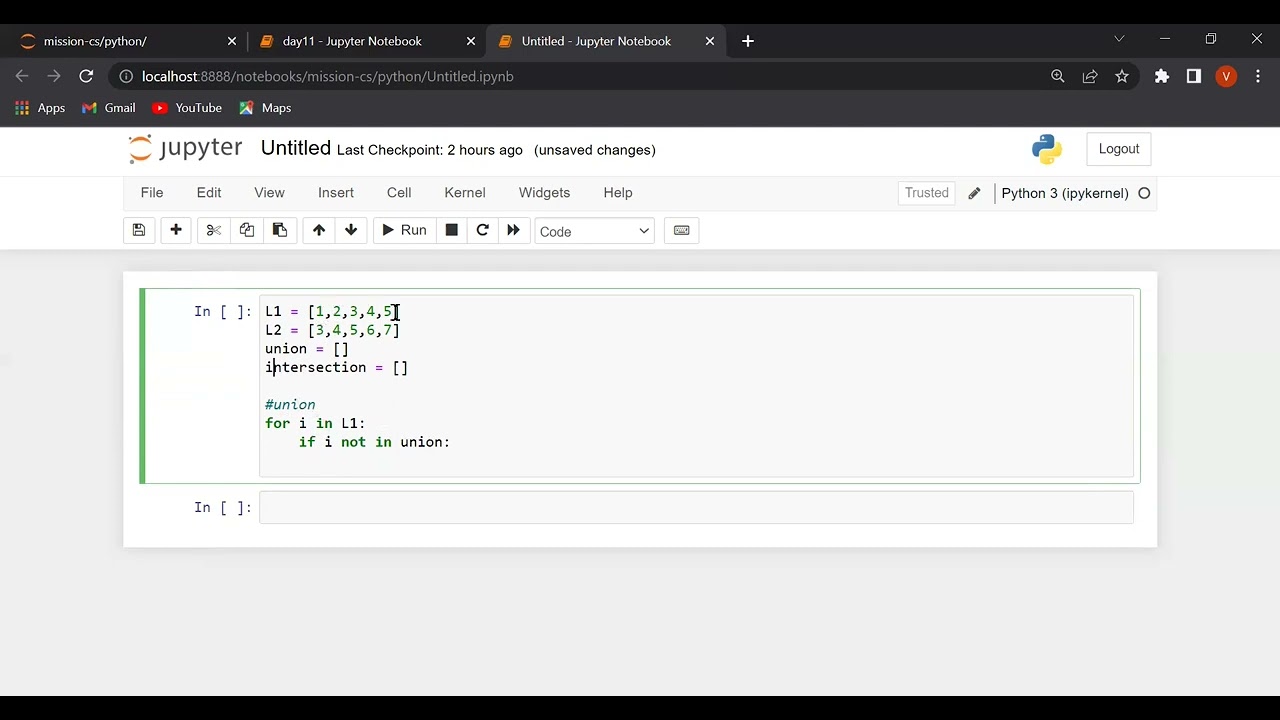
Python program to find union and intersection of two lists. YouTube
An intersection in python can be performed between two or more lists or sets. With intersection, we get the common elements present in all the lists, i.e., it returns the elements which exist in all the lists. Example : If we have two lists - A and B containing the following elements: 1 2 3 A = [0,1,10,5,8] B = [7,1,3,11,0]
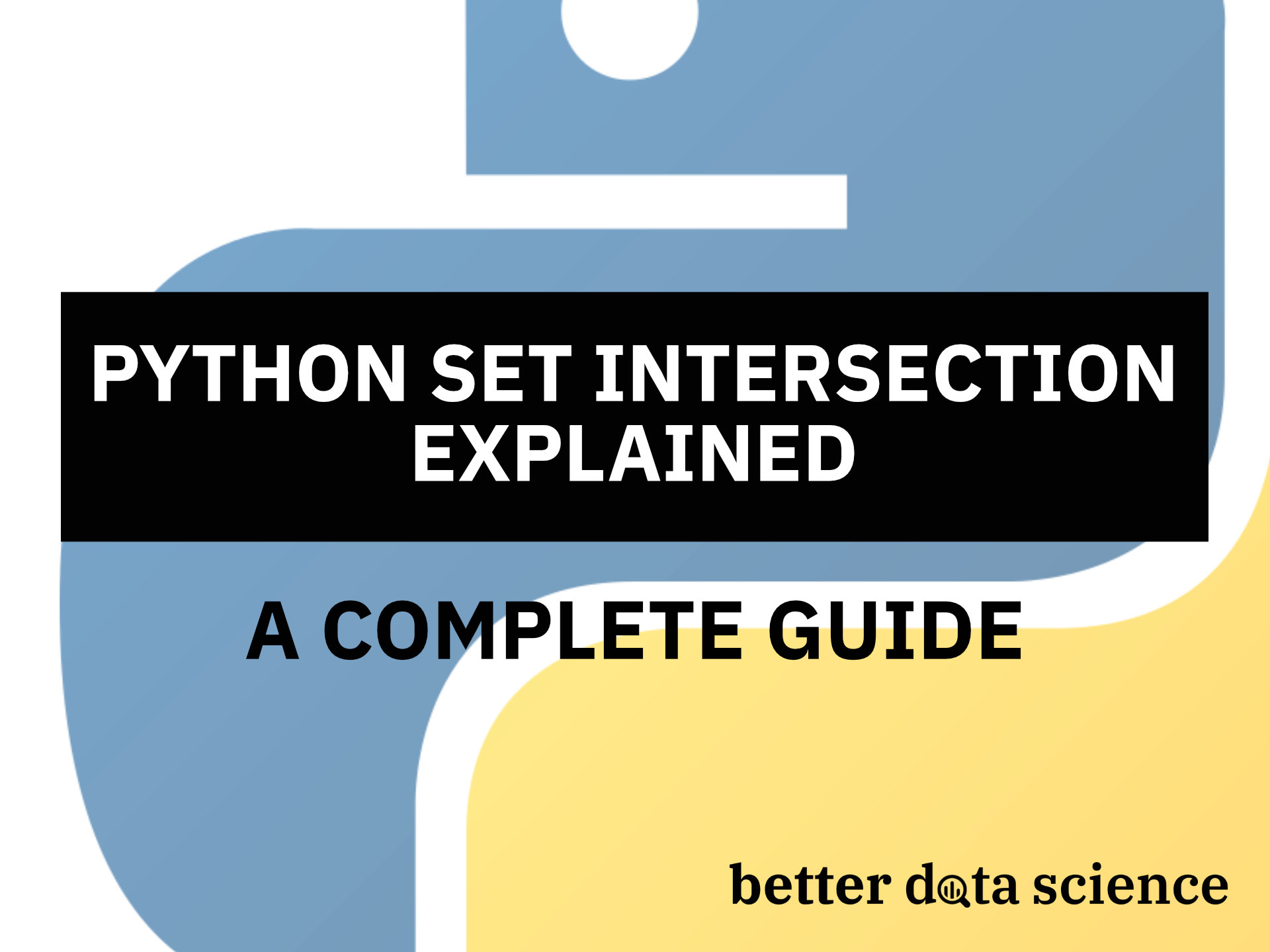
Python Set Intersection The Ultimate Guide for Beginners Better Data Science
Python 3 provides various built-in methods to find the intersection of two lists. In this article, we will discuss the different ways to find the intersection of two lists in Python 3 and provide 5 code snippets for usage examples. Using the set() Function. The easiest way to find the intersection of two lists in Python 3 is to use the set.
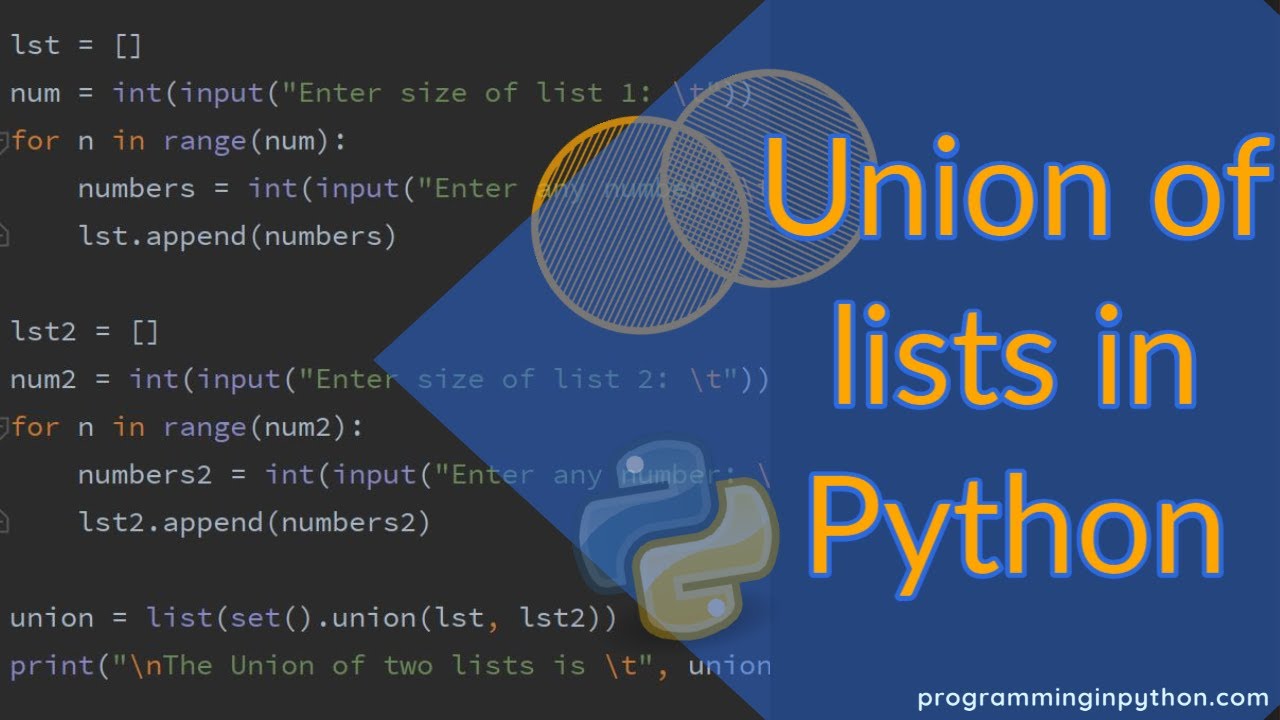
Python Program To Find Intersection Of Two Lists BuzzFeedNews
Because the number [3] is common in both lists declared. The ways to find an intersection of two lists are listed below: Using Counter() Method; Using Loop Method; Using Set() Method; Let us find out each method with one sample example & its output. Method 1: Find Out The Intersection Of Two Lists Using Counter() Method
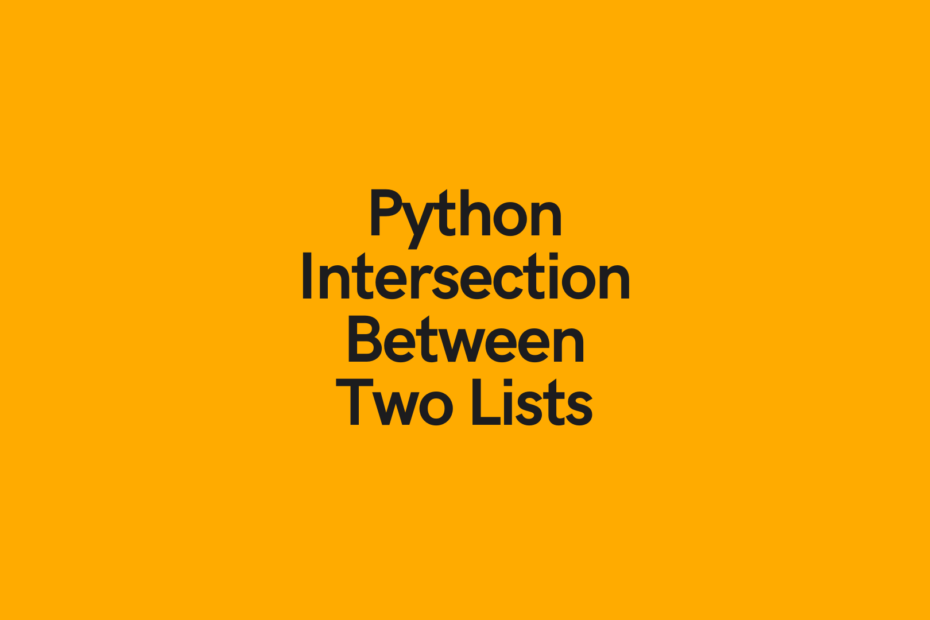
Python Intersection Between Two Lists โข datagy
Example intersection of two lists in Python. A simple example code finds the intersection of list1 and list2. list1 = [1, 2, 3] list2 = [1, 3, 5] res = set.intersection (set (list1), set (list2)) print (list (res)) The & operator can only be used to find the intersection of two sets, not lists.
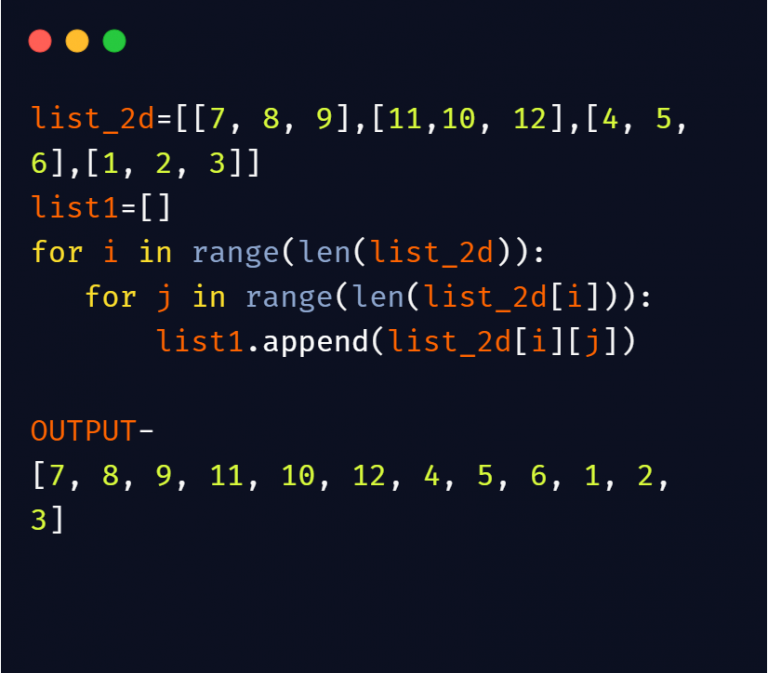
Python 2d List From Basic to Advance Python Pool
Lists are data structures that store multiple variables or objects in a single variable. You can append, or remove elements from a single list. Suppose you have two lists and want to find the intersection of these two how you will do so. In this post, you will learn the various methods to find the Intersection of Two Lists in Python.